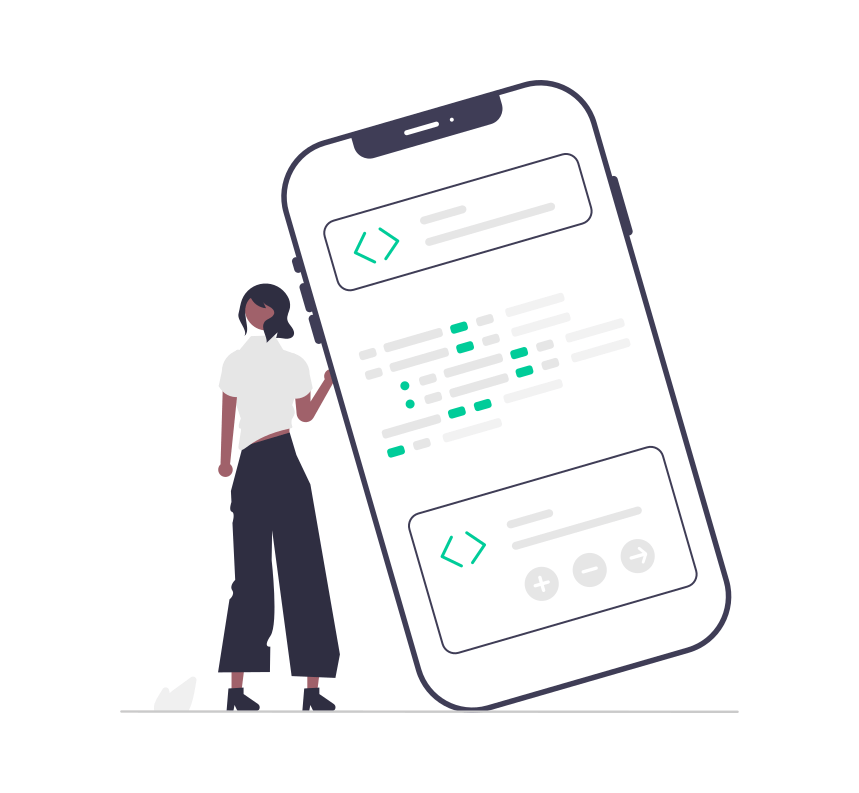
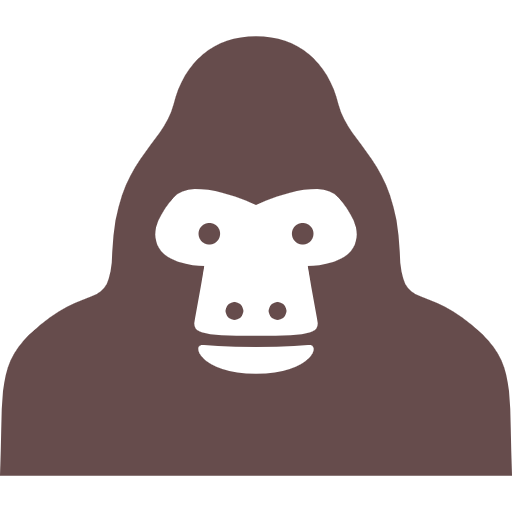
そんな悩みにお答えします。
モーダルウィンドウの実装はWeb制作をする上では欠かせないスキルです。
そこでやり方は2つあります。
プラグインを使う方法と使わない方法です。
今回はプラグインを使わずに実装する方法を紹介します。
さらにdata関数を使った方法で行います。
慣れればコピペでいいとは思いますが、一度は自分の手でひととおりコードを書いてみましょう。
目次
モーダルウインドウとは?
モーダルとは「モードを持つ」という意味で「あなたがこのウィンドウを閉じるまでなにもさせません」というウィンドウのことです。
ウィンドウ内で表示された操作を完了する、あるいはキャンセルするかしてそのウインドウを閉じない限り、親ウインドウに対する操作はできません。
モーダルウインドウは画像をクリックしたら拡大表示されたり、コンテンツをポップアップさせたりします。
今回はボタンをクリックしたらテキストが表示されるというのを作ります。
HTML
<div class="modal-open-button">
<a class="js-open-button" href="" data-target=".target-modal">open</a>
</div>
<div class="modal-contact target-modal">
<div class="modal-contact-head">見出し</div>
<div class="modal-contact-content">
<div class="modal-contact-title">タイトル1</div>
<div class="modal-contact-text">テキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキスト</div>
<div class="modal-contact-title">タイトル2</div>
<div class="modal-contact-text">テキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキスト</div>
<div class="modal-contact-title">タイトル3</div>
<div class="modal-contact-text">テキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキスト</div>
<div class="modal-contact-title">タイトル4</div>
<div class="modal-contact-text">テキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキスト</div>
</div>
<div class="modal-contact-button">
<a class="js-close-button" href="" data-target=".target-modal">閉じる</a>
</div>
<div class="modal-contact-icon">
<a class="js-close-button" href="" data-target=".target-modal"><img src="img/02.png" alt=""></a>
</div>
</div>
<div class="modal-contact-background target-modal"></div>
「modal-contact」「modal-contact-background」の部分がボタンをクリックしたら表示される部分です。
「js-open-button」「js-close-button」「data-target=”.target-modal”」についてはあとで説明します。
CSS
.modal-contact {
position: fixed;
z-index: 20;
width: 800px;
max-width: calc(100% - 20px * 2);
height: 700px;
max-height: calc(100% - 20px * 2);
background: #fff;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
padding: 50px;
color: #707070;
display: none;
}
.modal-contact-head {
font-size: 24px;
font-weight: bold;
text-align: center;
padding-bottom: 30px;
position: relative;
}
.modal-contact-head:after {
content: '';
width: 100px;
height: 3px;
position: absolute;
left: 50%;
bottom: 0;
transform: translateX(-50%);
background: #00CC99;
}
.modal-contact-content {
margin-top: 40px;
height: calc(100% - 150px);
overflow: auto;
}
.modal-contact-content > :first-child {
margin-top: 0;
}
.modal-contact-title {
font-size: 18px;
font-weight: bold;
margin-top: 40px;
}
.modal-contact-text {
margin-top: 30px;
}
.modal-contact-button {
margin-top: 20px;
text-align: right;
}
.modal-contact-button a {
display: inline-block;
width: 150px;
padding: 10px;
text-align: center;
color: #fff;
background: #00CC99;
font-weight: bold;
border-radius: 5px;
box-shadow: 0 4px 7px rgba(0,0,0,0.2);
}
.modal-contact-icon {
position: absolute;
width: 30px;
height: 30px;
top: -20px;
right: -15px;
z-index: 30;
}
.modal-contact-background {
position: fixed;
top: 0;
left: 0;
width: 100%;
height: 100%;
background: rgba(0,0,0,0.3);
z-index: 10;
display: none;
}
「modal-contact」「modal-contact-background」は最初の時点では表示させないのでdisplay: none;としておきます。
jQuery
$(function() {
$('.js-open-button').on('click', function(e) {
e.preventDefault();
var target = $(this).data('target');
$(target).fadeIn();
});
$('.js-close-button').on('click', function(e) {
e.preventDefault();
var target = $(this).data('target');
$(target).fadeOut();
});
});
それではjQueryを書いていきます。
クリックイベントを設定するには次の書き方でしたね。
$('[対象の要素]').on('click', function() {
/* 処理内容 */
});
- モーダルウインドウで表示する要素(最初は見えない)はdisplay: none;にする
- ターゲットの要素がクリックされたら、jQueryのfadeIn()メソッド(あるいはshow()メソッド)で表示する
- 閉じる用のターゲットがクリックされたら、jQueryのfadeOut()メソッド(あるいはhide()メソッド)で非表示にする
- fadeIn()はその場でフワッと表示、show()は上から下に向かってアニメーションしながら表示
- fadeOut()はその場でフワッと消える、hide()は上にスライドしながら消えていく
ターゲットの要素がクリックされたら、jQueryのfadeIn()メソッドで表示する
まずクリックされるターゲット要素は「 .js-open-button 」になります。
さらにaタグ本来の動きを無効化させるためのコードを足すとこうなります。
$('.js-open-button').on('click', function(e) {
e.preventDefault();
次にdata関数を使います。
主にjQueryなどで値を取得したり変更したりできる属性のことです
data-〇〇とし、対象となる要素のクラス名やidを入れます。
今回の場合だと「 data-target=”.target-modal” 」としています。
これは data-targetに”.target-modal”という値を設定した ことになります。
<div class="modal-open-button">
<a class="js-open-button" href="" data-target=".target-modal">open</a>
</div>
そして 「data-target」と同じクラスを「modal-contact」と「modal-contact-background」につけておきます。
<div class="modal-contact target-modal">
<div class="modal-contact-background target-modal">
$(this).data('target')
「this」は「js-open-button」なのでaタグの要素を取得します。
さらにdata-〇〇の部分の〇〇をdata(‘〇〇’)と入れます。
これで 「data-target=”.target-modal”」の値 が取得できるようになります。
それではきちんと値が取れているか確認してみます。
$('.js-open-button').on('click', function(e) {
e.preventDefault();
console.log($(this).data('target'));
});
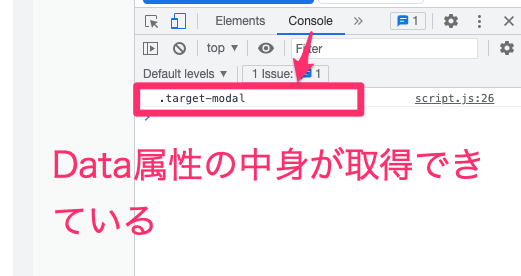
var target = $(this).data('target');
変数に格納します。
$(target).fadeIn();
最後にopenボタンをクリックしたときの挙動なのでfadeIn()とします。
結果以下のようになります。
$('.js-open-button').on('click', function(e) {
e.preventDefault();
var target = $(this).data('target');
$(target).fadeIn();
});
同じやり方で「閉じる用のターゲットがクリックされたら、jQueryのfadeOut()メソッドで非表示にする」も書いてみるとこのようになります。
$('.js-close-button').on('click', function(e) {
e.preventDefault();
var target = $(this).data('target');
$(target).fadeOut();
});
これでモーダルウインドウの実装ができました。
全体の挙動は次のようになります。
data属性のメリット
jQueryで直接クラス名を指定してコードを書いた場合、仮にHTML側でそのクラス名を変えるとなるとHTML、jsの2つに変更を加える必要があります。
しかしdata属性を使えばHTMLにてdata-targetに指定した値を書き換えるだけで済みます。
jsのコードは変更する必要がありません。
コードの保守性という点ではメリットは大きいです。
まとめ:モーダルウインドウの作り方を覚えよう!
今回のdata属性を使う方法は初心者にはなかなか敷居が高いです。
ですがコードの効率性や保守性を考えれば、習得しておくべき手順です。
ぜひ覚えておきましょう。
投稿者 トシ
コメントを残す